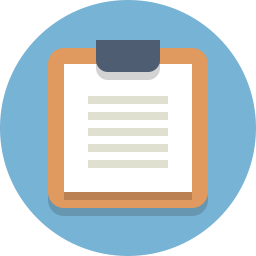
Lesson Plan
Review
- Previous week
- Critical think based on previous question
Step 1: The Hardware (15 minutes)
Our sonar sensor is going to give our robot the ability to sense the world around it. What we have been working with up to this point, our LEDs, motors, buzzers, etc. have been what we call outputs. This means that the component is meant to function by sending an electrical signal to the component from the robot’s brain. The sonar sensor however, is what’s known as an input. This means that our robot will receive a signal from the component rather than sending one to to it. I like to say that an output component listens and an input component talks.
What you see below is a picture of our HC-SRO4 sonar sensor;
Almost looks like it’s staring at you, huh? The sensor board notably has two wide eyed speakers among its notable parts. On its backside it has a lot of parts you may be familiar with: resistors, capacitors, ICs and the like. Fortunately we don’t need to go into detail about those parts to operate this sensor. The only thing we need to worry about are those speakers and the four pins extruding from the bottom of the board.
Those four pins are labeled Vcc, Trig, Echo and GND. The pin labeled GND, unsurprisingly must be connected to the GND pin on our Uno board, and I recommend doing this rst, as connecting the Vcc pin beforehand can have unexpected side effects on the sonar sensor. Speaking of, you may be wondering what the Vcc pin is. Well, for reasons I will not explain here the Vcc pin is the voltage pin of the sensor, meaning that it will be connected to 5V through our Uno board.
That leaves only the Trig and Echo pins to explain, but before I do that I would like to make a confession. Earlier I told you that the sonar sensor is an input component and explicitly told you that meant it would only talk to the Uno board, not listen. It turns out that the sonar sensor needs to both talk and listen, or send and receive signals, in order to function. So if it does both is it a input or output component? or some type of hybrid? Well, we still like to think of it as an input component because it’s main job is talking, we think the information it sends back to us is more important than the signals we give to it.
Knowing this let’s get back to our two remaining pins. The Trig pin is the output pin, it is going to listen for signals we give it. with this in mind we will need to connect it to one of the programmable pins on the Uno board. That pin will later be programmed as an output. The Echo pin is the input pin, and we will be listening for the signals it gives us. The Echo pin will be connected to another programmable pin on the Uno board which will later be programmed as an input.
The Science
So for now, we know all we need to know about the hardware and are ready to dive into the physics of this sensor works. First of all, I’d like to go over how the sonar sensor sends and receives signals. Below is a diagram of how our sonar system works.
We are utilizing a very handy bit of physics knowledge to use our sensors; The speed of sound in air is always about the same, 340 meters/second. For those of you who are mathematically inclined the distance something has travelled is equal to the speed it is travelling multiplied by the time it has been travelling; Distance = speed x time. Because we are fully aware of the speed of the sound wave we are creating we can alter that equation; Distance = 340m/s x time. From that equation we know that the only way the distance of the object changes is if the time is has been travelling changes.
In addition the sound wave needs to travel to the object, bounce off of the object, then travel back to the sensor. So the distance that the sound wave travels is actually twice the distance between the sensor and the object. For that reason the equation describing the distance read by the sensor is as follows;
2 x Distance = 340m/s x time {:class=”text-center “}
This is the equation we must consider in our code for the sensor to behave appropriately. If you are using Ardublock the math is inside the ultrasonic block already.
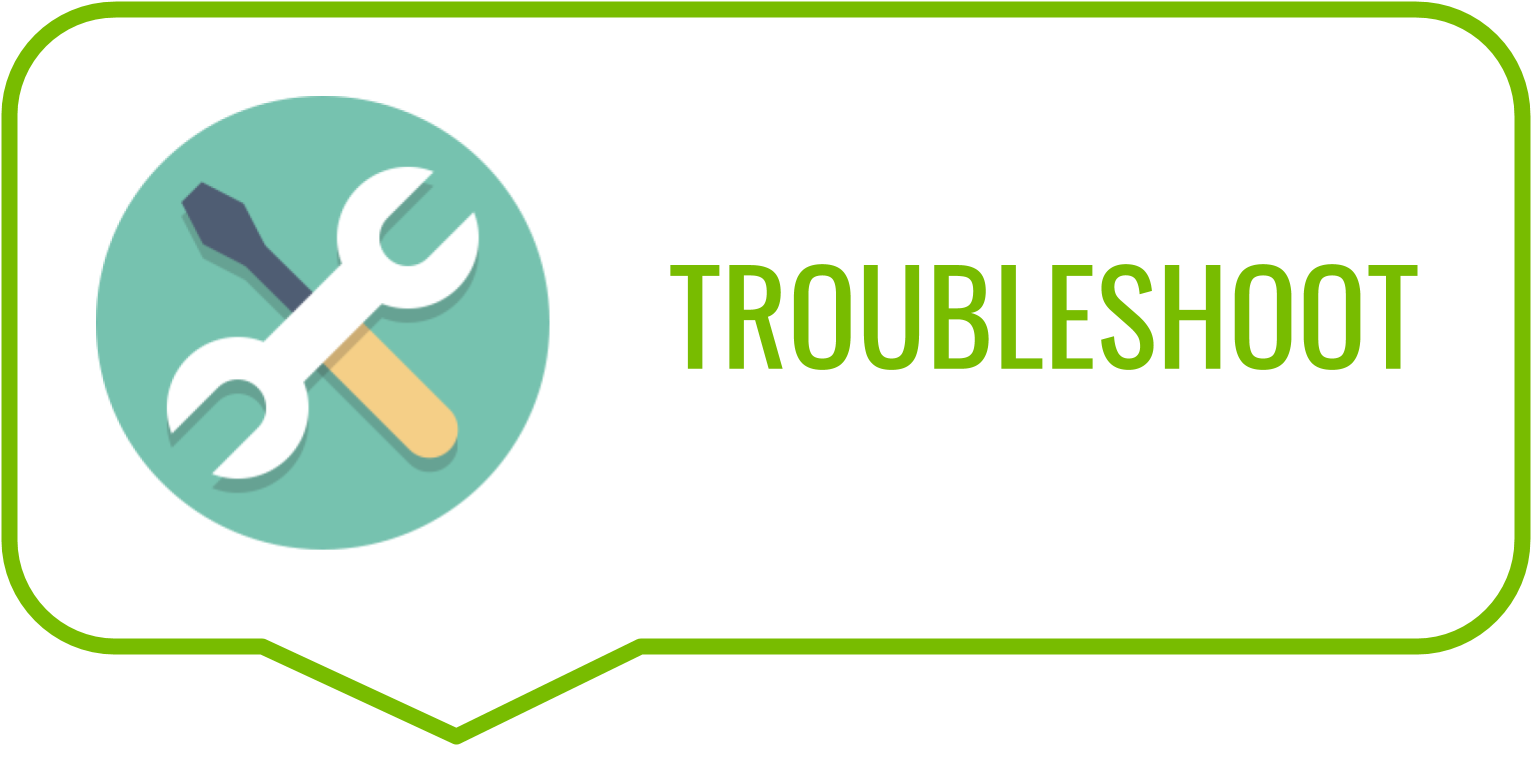
Step 2: Wiring The Ultrasonic Sensor
Placing Your Hardware
Before wiring your sensor, you’ll want to place your sensor onto your breadboard. See the picture below. Notice that the pins are aligned in a way that they are each on different rows. This is important because each pin needs to go to a different signal. You also want to make sure that your sensor is facing outward on the front. If you need to reposition your breadboard to do so, go ahead and do that.
FRONT VIEW
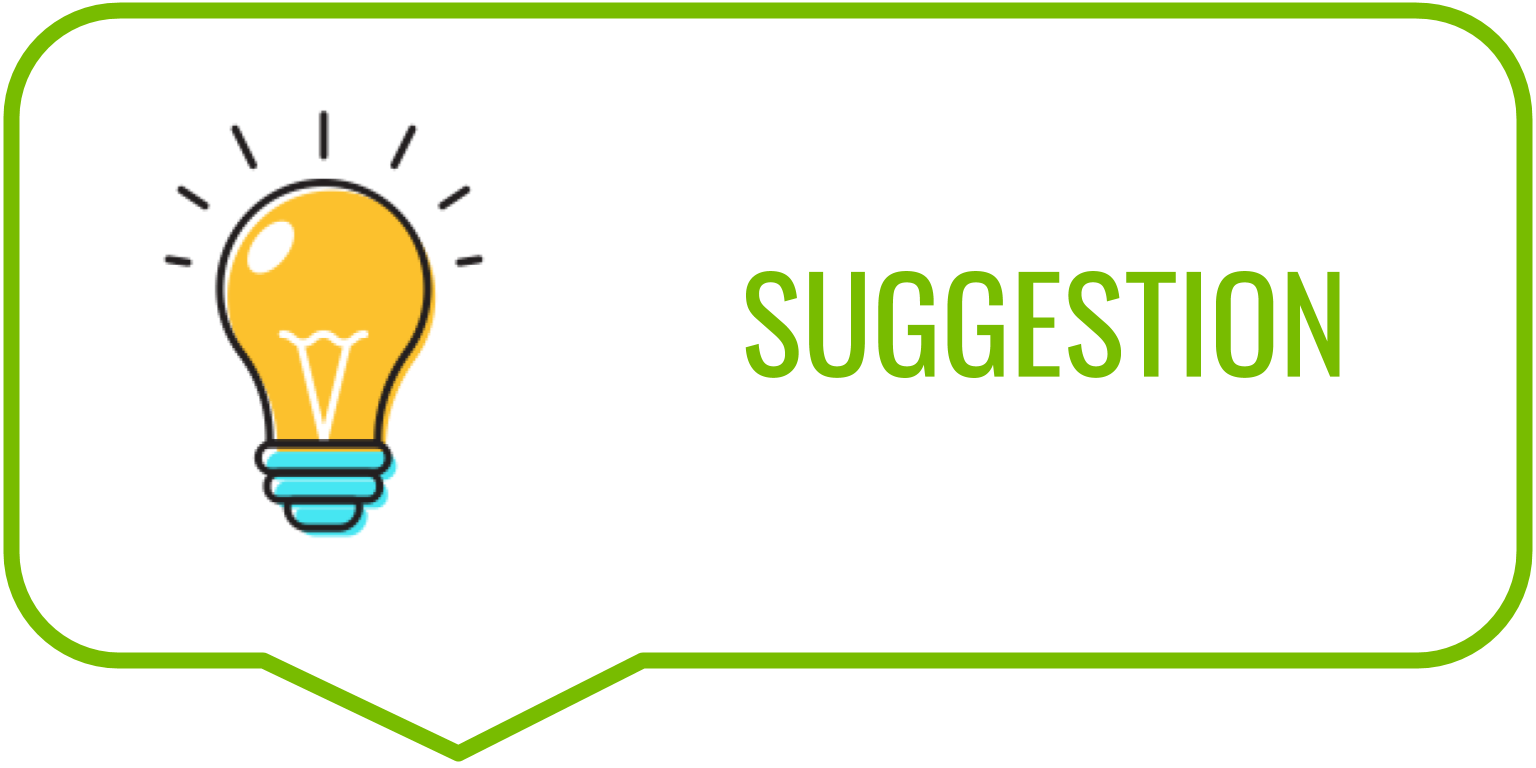
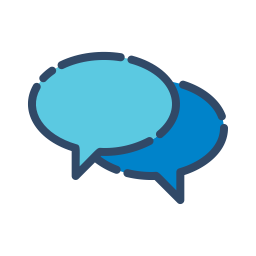
REFLECTION
Comprehension
- What are we assessing?
Challenges
- What was hard in today's class?
Enjoyment
- What was fun about today's class?
Mindset
- What did you learn about yourself?
- What would you like to improve?
Community
- How can what you learned impact those around you?